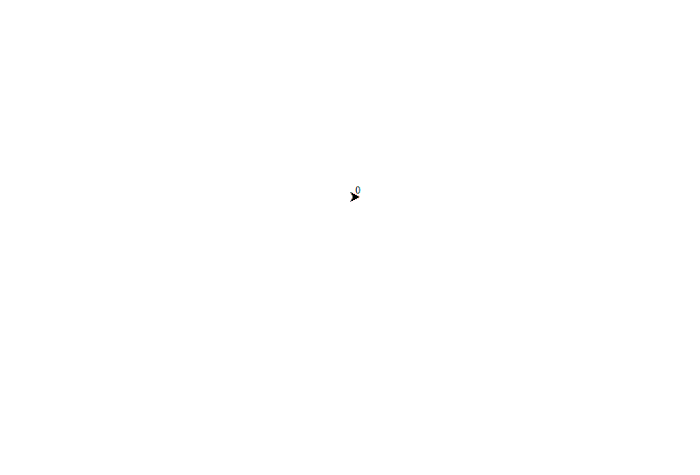
I’m going to make turtles compete with each other by using the Python library turtle.
You can do a lot of things with turtles like the GIF image above.
The environment and code
OS: Microsoft Windows 10 Pro
Pyhton version:3.10
from turtle import *
from random import randint
import time
#Lines
for step in range(11):
write(step, align='center')
speed(80)
right(90)
forward(10)
pendown()
forward(150)
penup()
backward(160)
left(90)
forward(20)
time.sleep(1)
#Red turtle
red = Turtle()
red.color('red')
red.shape('turtle')
red.penup()
red.goto(0, -10)
#Blue turtle
blue = Turtle()
blue.color('blue')
blue.shape('turtle')
blue.penup()
blue.goto(0, -30)
#Yellow turtle
yellow = Turtle()
yellow.color('orange')
yellow.shape('turtle')
yellow.penup()
yellow.goto(0, -60)
#Green turtle
green = Turtle()
green.color('green')
green.shape('turtle')
green.penup()
green.goto(0, -90)
#Black turtle
black = Turtle()
black.color('black')
black.shape('turtle')
black.penup()
black.goto(0, 60)
#Gray turtle
gray = Turtle()
gray.color('gray')
gray.shape('turtle')
gray.penup()
gray.goto(0, -120)
#Pink turtle
pink = Turtle()
pink.color('pink')
pink.shape('turtle')
pink.penup()
pink.goto(0, -150)
time.sleep(3)
#Moving each turtles x100
for kame in range(100):
red.forward(randint(1, 2))
blue.forward(randint(-1, 3))
yellow.forward(randint(-3, 4))
green.forward(randint(-4, 5))
black.right(90)
gray.forward(randint(-3, -2))
pink.forward(randint(-10, 10))
Explanation of the code
Import each library.
Used the random library to make the turtles randomly.
Time library for making turtles wait for a bit before the race, so this one isn’t necessary.
from turtle import *
from random import randint
import time
Starting at 0, repeat with a for loop until 10 lines are drawn as the racetrack.
for step in range(11):
write(step, align='center')
speed(80)
right(90)
forward(10)
pendown()
forward(150)
penup()
backward(160)
left(90)
forward(20)
Specify the colors of turtles with color().
You can use fillcolor() to specify more colors.
The shape() is used to specify the shape of the turtles and goto() is for specifying the coordinates of the first placement.
You can adjust the rest of the turtles while debugging.
#Red turtle
red = Turtle()
red.color('red')
red.shape('turtle')
red.penup()
red.goto(0, -10)
Make them move 100 times with a for loop.
For each turtle, one of the numbers from (number, number) is randomly selected.
I didn’t do it this time, but it will be better if you can display something like “GOAL!” at the end of the race.
for kame in range(100):
red.forward(randint(1, 2))
blue.forward(randint(-1, 3))
yellow.forward(randint(-3, 4))
green.forward(randint(-4, 5))
black.right(90)
gray.forward(randint(-3, -2))
pink.forward(randint(-10, 10))
Originally, the turtle was a descriptive library, but now they added Turtle Shape so that it can be used in this way.
(Check the official document for more details.)
I think it's good practice for beginners to learn Python.
This blog post is translated from a blog post written by Kawa Ken on our Japanese website Beyond Co..